I simply created a Jquery slidedown effect for the Login form and a hover search box. Very basic and easy to make.
Here is the CSS.
Here is the HTML
Here is the Jquery
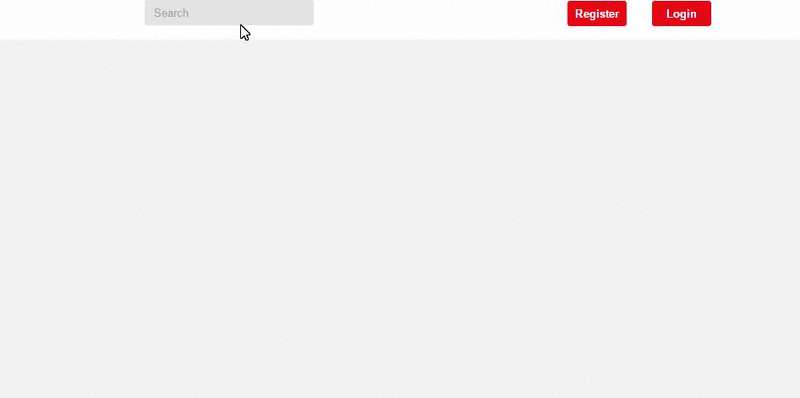
Here is the CSS.
Code:
body {
margin:0;
background-color:#f2f2f2;
}
#menu1 input[name="register"] {
background-color: red;
border: 1px solid red;
color:white;
font-weight: bold;
height: 30px;
width:280px;
border-radius:3px;
margin-top:120px;
margin-left: 10px;
cursor: pointer;
}
#menu, #menu1{
height: 60px;
background-color: white;
}
#menu1 {
background-color: white;
height: 300px;
margin-top: 50px;
box-shadow: 0 3px 3px #ccc;
border-radius: 3px;
margin-left:500px;
width: 300px;
display: none;
}
#menu input[type="text"] {
background-color: #e4e4e4;
border: 1px solid #e4e4e4;
height: 30px;
margin-top: 13px;
width:200px;
margin-left:500px;
outline:0;
text-indent: 10px;
border-radius: 3px;
}
#menu input[type="text"]:hover {
border:1px solid #e50914;
}
#menu button[class="login"] {
background-color:#e50914;
position:absolute;
border: 1px solid #e50914;
height: 30px;
width: 70px;
margin-top:14px;
margin-left:1100px;
border-radius: 3px;
outline:0;
cursor:pointer;
color:white;
font-weight: bold;
}
#menu button[class="register"] {
background-color:#e50914;
position:absolute;
border: 1px solid #e50914;
height: 30px;
width: 70px;
margin-top:14px;
margin-left:1000px;
border-radius: 3px;
outline:0;
cursor:pointer;
color:white;
font-weight: bold;
}
Here is the HTML
Code:
<html>
<title>Search</title>
<head></head>
<link rel="stylesheet" type="text/css" href="css/style.css">
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<script src="js/menu.js"></script>
<body>
<div id="menu">
<button class="login">Login</button>
<button class="register">Register</button>
<input type="text" name="search" placeholder="Search" />
</div>
<div id="menu1">
<form>
<input type="text" name="username" placeholder="Username">
<input type="text" name="Email" placeholder="Email">
<input type="password" name="password" placeholder="Password">
<input type="submit" name="register" value="Register">
</form>
</div>
</body>
</html>
Here is the Jquery
Code:
$(document).ready(function(){
$("#menu button").click(function(){
$("#menu1").slideDown(250);
});
});